C:\Users\Student13\Documents\DEVELOPER\CODE BLOCKS\Components\Teste\main.cpp
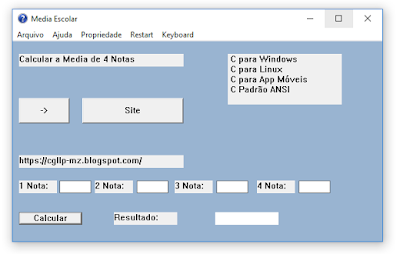
#if defined(UNICODE) && !defined(_UNICODE)
#define _UNICODE
#elif defined(_UNICODE) && !defined(UNICODE)
#define UNICODE
#endif
#include <tchar.h>
#include <windows.h>
#include <math.h>
#include <stdio.h>
#include <memory.h>
#define BTN1 1
#define BTN2 2
#define BTN3 3
static HWND nome, nome2, num1, num2, num3, num4, resultado;
LRESULT CALLBACK WindowProcedure (HWND, UINT, WPARAM, LPARAM);
void addMenus(HWND);
HMENU hMenu;
TCHAR szClassName[ ] = _T("CodeBlocksWindowsApp");
int WINAPI WinMain (HINSTANCE hThisInstance, HINSTANCE hPrevInstance, LPSTR lpszArgument, int nCmdShow) {
HWND hwnd; /* This is the handle for our window */
MSG messages; /* Here messages to the application are saved */
WNDCLASSEX wincl; /* Data structure for the windowclass */
wincl.hInstance = hThisInstance;
wincl.lpszClassName = szClassName;
wincl.lpfnWndProc = WindowProcedure; /* This function is called by windows */
wincl.style = CS_DBLCLKS; /* Catch double-clicks */
wincl.cbSize = sizeof (WNDCLASSEX);
wincl.hIcon = LoadIcon (NULL, IDI_QUESTION);
wincl.hIconSm = LoadIcon (NULL, IDI_QUESTION);
wincl.hCursor = LoadCursor (NULL, IDC_ARROW);
wincl.lpszMenuName = NULL; /* No menu */
wincl.cbClsExtra = 0; /* No extra bytes after the window class */
wincl.cbWndExtra = 0; /* structure or the window instance */
wincl.hbrBackground = GetSysColorBrush(COLOR_ACTIVECAPTION);
/* Register the window class, and if it fails quit the program */
if (!RegisterClassEx (&wincl))
return 0;
hwnd = CreateWindowEx (
0, szClassName, _T("Media Escolar"), WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT, CW_USEDEFAULT, 600, 375,
HWND_DESKTOP, NULL, hThisInstance, NULL
);
/* Make the window visible on the screen */
ShowWindow (hwnd, nCmdShow);
/* Run the message loop. It will run until GetMessage() returns 0 */
while (GetMessage (&messages, NULL, 0, 0)) {
TranslateMessage(&messages);
DispatchMessage(&messages);
}
return messages.wParam;
}
LRESULT CALLBACK WindowProcedure (HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam) {
switch(message) {
case WM_CREATE:
addMenus(hwnd);
nome = CreateWindowW(L"static", L"Calcular a Media de 4 Notas",
WS_VISIBLE | WS_CHILD,
10, 20, 260, 20,
hwnd, NULL, NULL, NULL);
CreateWindow(TEXT("button"), TEXT(" -> "), WS_VISIBLE | WS_CHILD,
10, 90, 80, 40, hwnd, (HMENU) BTN1, NULL, NULL);
CreateWindow(TEXT("button"), TEXT("Site"), WS_VISIBLE | WS_CHILD,
110, 90, 160, 40,
hwnd, (HMENU) BTN2, NULL, NULL);
nome2 = CreateWindowW(L"static", L"https://cgllp-mz.blogspot.com/",
WS_VISIBLE | WS_CHILD,
10, 180, 260, 20,
hwnd, NULL, NULL, NULL);
CreateWindow(TEXT("static"), " C para Windows\n C para Linux\n C para App Móveis\n C Padrão ANSI", WS_VISIBLE | WS_CHILD,
340, 20, 180, 80,
hwnd, NULL, NULL, NULL);
CreateWindowW(L"static", L"1 Nota: ",
WS_VISIBLE | WS_CHILD,
10, 220, 60, 20,
hwnd, NULL, NULL, NULL);
num1 = CreateWindow(TEXT("edit"), TEXT(""), WS_VISIBLE | WS_CHILD | WS_BORDER,
74, 220, 50, 20,
hwnd, NULL, NULL, NULL);
CreateWindowW(L"static", L"2 Nota: ",
WS_VISIBLE | WS_CHILD,
130, 220, 60, 20,
hwnd, NULL, NULL, NULL);
num2 = CreateWindow(TEXT("edit"), TEXT(""), WS_VISIBLE | WS_CHILD | WS_BORDER,
196, 220, 50, 20,
hwnd, NULL, NULL, NULL);
/**Cópia*/
CreateWindowW(L"static", L"3 Nota: ",
WS_VISIBLE | WS_CHILD,
256, 220, 60, 20,
hwnd, NULL, NULL, NULL);
num3 = CreateWindow(TEXT("edit"), TEXT(""), WS_VISIBLE | WS_CHILD | WS_BORDER,
322, 220, 50, 20,
hwnd, NULL, NULL, NULL);
CreateWindowW(L"static", L"4 Nota: ",
WS_VISIBLE | WS_CHILD,
386, 220, 60, 20,
hwnd, NULL, NULL, NULL);
num4 = CreateWindow(TEXT("edit"), TEXT(""), WS_VISIBLE | WS_CHILD | WS_BORDER,
452, 220, 50, 20,
hwnd, NULL, NULL, NULL);
CreateWindow(TEXT("button"), TEXT("Calcular"), WS_VISIBLE | WS_CHILD | WS_BORDER,
10, 270, 100, 20,
hwnd, (HMENU) BTN3, NULL, NULL);
CreateWindow(TEXT("static"), TEXT("Resultado:"), WS_VISIBLE | WS_CHILD,
160, 270, 100, 20,
hwnd, NULL, NULL, NULL);
resultado = CreateWindow(TEXT("edit"), TEXT(""), WS_VISIBLE | WS_CHILD,
320, 270, 100, 20,
hwnd, NULL, NULL, NULL);
break;
case WM_COMMAND:
if(LOWORD(wParam)==BTN1) {
int len = GetWindowTextLength(nome)+1;
static char title[500];
GetWindowText(nome, title, len);
MessageBox(NULL, title, "", MB_OK);
return 0;
}
if(LOWORD(wParam)==BTN2) {
int com = GetWindowTextLength(nome)+1;
static char titulo[500];
GetWindowText(nome2, titulo, com);
MessageBox(NULL, titulo, "", MB_OK);
return 0;
}
if(LOWORD(wParam)==BTN3) { /**Quando clicamos em calcular*/
int n1 = GetWindowTextLength(num1)+1;
int n2 = GetWindowTextLength(num2)+1;
int n3 = GetWindowTextLength(num3)+1;
int n4 = GetWindowTextLength(num4)+1;
static char t1[50], t2[50], t3[50], t4[50];
GetWindowText(num1, t1, n1);
GetWindowText(num2, t2, n2);
GetWindowText(num3, t3, n3);
GetWindowText(num4, t4, n4);
float res = (atof(t1)+atof(t2)+atof(t3)+atof(t4))/4;
char tam[30];
sprintf(tam, "%.2f", res);
//ltoa(res, tam, 10);
SetWindowText(resultado, tam);
FILE *arq;
arq=fopen("Teste.txt", "w+");
fwrite(tam, sizeof(arq),10,arq);
fclose(arq);
}
break;
case WM_DESTROY:
PostQuitMessage (0); /* send a WM_QUIT to the message queue */
break;
default: /* for messages that we don't deal with */
return DefWindowProc (hwnd, message, wParam, lParam);
}
return 0;
}
void addMenus(HWND hwnd) {
hMenu = CreateMenu();
HMENU hFileMenu = CreateMenu();
AppendMenu(hMenu, MF_STRING, NULL, "Arquivo");
AppendMenu(hMenu, MF_STRING, NULL, "Ajuda");
AppendMenu(hMenu, MF_STRING, NULL, "Propriedade");
AppendMenu(hMenu, MF_STRING, NULL, "Restart");
AppendMenu(hMenu, MF_STRING, NULL, "Keyboard");
SetMenu(hwnd, hMenu);
}
Comentários
Postar um comentário